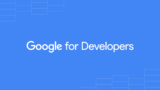
Quickstart: Run a Search Console App in Python | Search Console API | Google for Developers
公式のクイックスタートではAPIを利用して、Search Consoleのプロパティのリストを取得しています。私はクエリ、url、インプレッション、CTR、順位の取得までをやりたかったので実装してみました。
取得したデータはDataFrameに格納したので、あとはデータベースにいれたり、ファイルとして出力したりできます。
Pythonで実装
import httplib2
import pandas as pd
import time
from apiclient.discovery import build
from oauth2client.client import OAuth2WebServerFlow
# クライアントIDとクライアントシークレットのセット
CLIENT_ID = '1001952***********'
CLIENT_SECRET = '9ERAffYC***********'
# 固定
OAUTH_SCOPE = 'https://www.googleapis.com/auth/webmasters.readonly'
# 固定
REDIRECT_URI = 'urn:ietf:wg:oauth:2.0:oob'
# Run through the OAuth flow and retrieve credentials
flow = OAuth2WebServerFlow(CLIENT_ID, CLIENT_SECRET, OAUTH_SCOPE, REDIRECT_URI)
authorize_url = flow.step1_get_authorize_url()
print ('Go to the following link in your browser: ' + authorize_url)
code = input('Enter verification code: ').strip()
credentials = flow.step2_exchange(code)
__init__() takes at most 4 positional arguments (5 given)
Go to the following link in your browser: https://accounts.google.com/o/oauth2/v2/auth?client_id=1001952***********
Enter verification code: 4/AAAPRc2***********
# Create an httplib2.Http object and authorize it with our credentials
http = httplib2.Http()
http = credentials.authorize(http)
webmasters_service = build('webmasters', 'v3', http=http)
property_uri = 'https://hato.yokohama/'
# 検索条件のセット
set_date = '2018-07-15'
request = {
'startDate' : set_date,
'endDate' : set_date,
'dimensions' : ['query', 'page'],
'dimensionFilterGroups' : [{
'filters' : [{
'dimension' : 'country',
'expression' : 'jpn'
}]
}],
'rowLimit' : 6
}
# Google Search Cosole APIの実行
response = webmasters_service.searchanalytics().query(siteUrl=property_uri, body=request).execute()
# APIで取得したデータを指定
rows = response['rows']
# dataframe型にセット
df = pd.DataFrame(rows)
df = df.astype({'keys' : str})
df['keys'] = df['keys'].str.replace('[', '')
df['keys'] = df['keys'].str.replace(']', '')
tmp = pd.DataFrame(index = df.index, columns=[])
# expand=True : 分割した値をdataframeとして取得する
tmp = df['keys'].str.split(',', expand=True)
# queryとurlのセット
df['query'] = tmp[0].str.replace('¥', '')
df['url'] = tmp[1].str.replace('¥', '')
# クエリで検索した日付のセット
df = df.loc[:, ['query', 'url', 'clicks', 'impressions', 'ctr', 'position']]
df['date'] = set_date
# timestampのセット
time_stamp = time.strftime('%Y-%m-%d %H:%M:%S')
df['time_stamp'] = time_stamp
df
query | url | clicks | impressions | ctr | position | date | time_stamp | |
---|---|---|---|---|---|---|---|---|
0 | ‘kaggle tableau’ | ‘https://hato.yokohama/category/kaggle/’ | 0.0 | 1.0 | 0.0 | 24.0 | 2018-07-15 | 2018-07-24 22:06:26 |
1 | ‘one hot encoding 機械 学習’ | ‘https://hato.yokohama/one-hot-encoding/’ | 0.0 | 1.0 | 0.0 | 9.0 | 2018-07-15 | 2018-07-24 22:06:26 |
2 | ‘one hot encoding 機械 学習’ | ‘https://hato.yokohama/one-hot-encoding/#toc13’ | 0.0 | 1.0 | 0.0 | 9.0 | 2018-07-15 | 2018-07-24 22:06:26 |
コメント